• 4 min read
How to create Custom Artisan Commands in Laravel
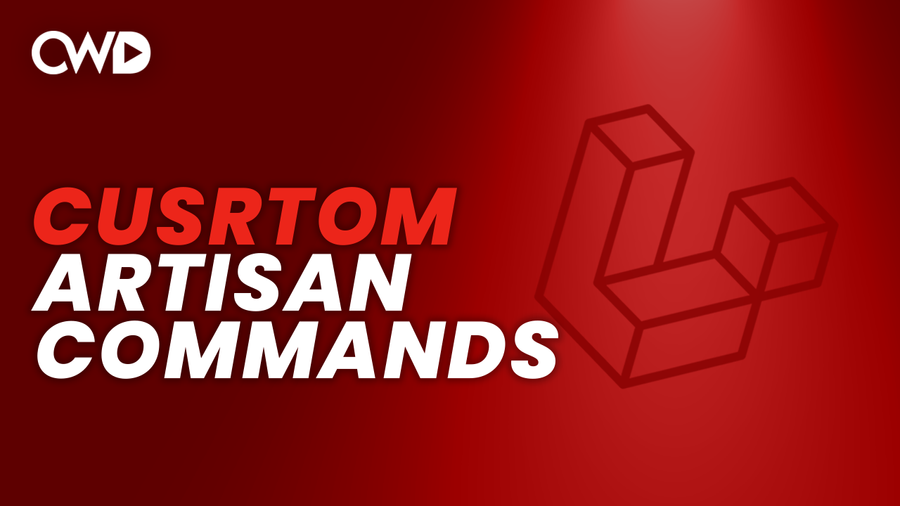
Artisan is the command line interface tool that Laravel uses. it is a script at the root of your project directory named artisan which will help you to perform tons of command line interface commands.
Before we get started with writing our custom artisan commands, we do need to create a model & migration to interact with the database.
php artisan make:model Product -m;
Open the products migration inside the /database/migrations folder and replace the up() method with with the following:
public function up()
{
Schema::create('products', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->double('original_price');
$table->tinyInteger('in_stock')->default(1);
$table->tinyInteger('status')->default(0);
$table->timestamps();
});
}
Obviously, don’t forget to migrate your tables :)
php artisan migrate
Create custom Artisan command
If you perform php artisan route:list command inside the CLI, you’ll find a complete list of Artisan commands that you can perform. If you scroll up to the make section, you’ll see that the third command is make:command, which will create a new artisan command for you.
Perform the following two commands to create two custom Artisan commands that we will be using in this tutorial:
php artisan make:command CreateNewProduct
php artisan make:command ShowAllProducts
Artisan allows you to add a $signature property directly in the command, by adding the following flag to it:
php artisan make:command CreateNewProduct --command=create:product
php artisan make:command ShowAllProducts --command=show:product
This will create two new classes inside the /app/Console/Commands directory called CreateNewProduct.php and ShowAllProducts.php. Let’s focus on the CreateNewProduct.php file first.
The $signature property will be the Artisan command that you need to run inside the CLI to use the command and the $description will be the description of the custom command. Let’s change it to the following:
protected $signature = 'create:product';
protected $description = 'Create a new product through Artisan';
Since we’re not working with an interface but within the CLI, we got to make sure that we ask the user for product data. This can be done through the ask() method.
public function handle()
{
$title = $this->ask('What is the product title?: ');
$original_price = $this->ask('What is the product price?: ');
$stock = $this->ask('Is the product in stock?: ');
$status = $this->ask('What is the product status?: ');
}
Once we have defined the questions, we need to make sure that we import the App\Models\Product since we’re going to use Eloquent to interact with our products table.
public function handle()
{
$title = $this->ask('What is the product title?: ');
$original_price = $this->ask('What is the product price?: ');
$stock = $this->ask('Is the product in stock?: ');
$status = $this->ask('What is the product status?: ');
Product::create([
'title' => $title,
'original_price' => $original_price,
'in_stock' => $stock,
'status' => $status
]);
$this->info('Product has been created!');
}
We have also created a new Custom artisan command inside /app/Console/Commands/ directory, which will show all products from the products table.
Once again, make sure that you change up the $signature and the $description of the ShowAllProducts.php class to the following:
protected $signature = 'show:product';
protected $description = 'Show all products through Artisan';
Instead of printing the output as an array, we’re going to use the table() method to output a simple ASCII table full of your data.
public function handle()
{
$headers = [
'id',
'title',
'original_price',
'in_stock',
'status',
'Created at',
'Updated at'
];
$data = Product::all()->toArray();
$this->table($headers, $data);
}
Run the following command inside the CLI to grab all products from the database:
php artisan show:product
Calling Artisan commands in normal code
There might be a case where you need to run your Artisan commands in either a controller of the web.php file. This can be done in two different ways. The easiest one, and in my opinion the most recommended one is using the Artisan Facade.
Inside your code, you can either use Artisan::call() or the Artisan::queue(). Both methods take one required and one optional parameter
- First parameter will be the terminal command [REQUIRED]
- Second parameter will be an array of parameters to pass it [OPTIONAL]
Artisan::call('show:product');
Artisan::queue('show:product');
Credits due where credits due…
Thanks to Laravel for giving me the opportunity to make this tutorial on Artisan Console.