• 4 min read
What is the difference between chunk and pagination in Laravel?
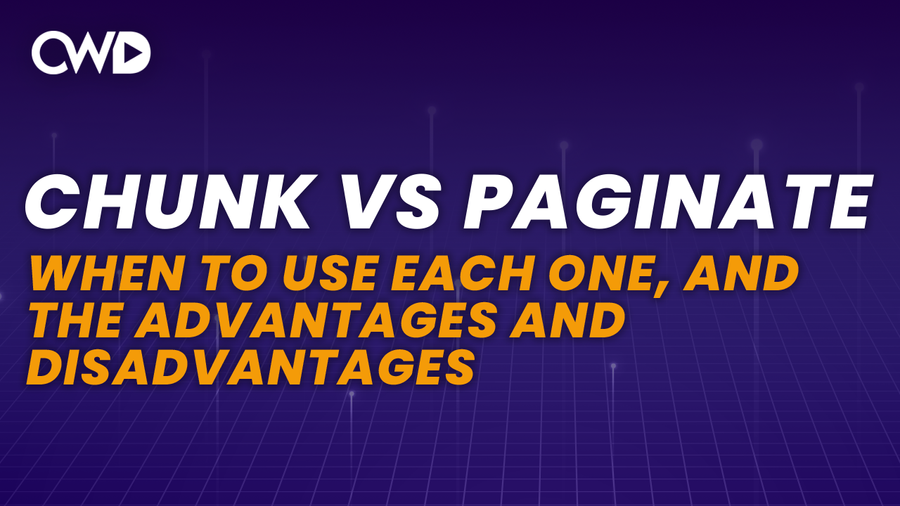
When it comes to working with large datasets in Laravel, you’ll often need to retrieve data from your database in a more efficient way. This is where Laravel’s chunk()
and paginate()
methods come into play. These methods allow you to retrieve large amounts of data from your database in smaller, more manageable chunks. In this blog post, we’ll explore the differences between these methods, when to use each one, and the advantages and disadvantages of each approach.
Chunk Method
The chunk()
method is used to retrieve a large amount of data from your database in smaller, more manageable chunks. This method is best used when you need to perform an operation on each record in the result set, or when you need to process the data in batches.
The chunk()
method retrieves a specified number of records (default is 100) and then passes them to a closure for processing. Once the closure has finished processing the records, the chunk()
method retrieves the next batch of records and continues the process until all records have been processed.
One advantage of using the chunk()
method is that it can help improve performance when working with large datasets. By retrieving records in smaller batches, you can avoid memory issues that can occur when working with large amounts of data. However, one disadvantage of using the chunk()
method is that it can be slower than the paginate()
method since it requires multiple database queries.
// Example of using the chunk method
DB::table('users')->orderBy('id')->chunk(100, function ($users) {
foreach ($users as $user) {
// Process each user
}
});
Paginate Method
The paginate()
method is used to retrieve a large amount of data from your database and display it in a paginated view. This method is best used when you need to display a large amount of data to the user in a user-friendly manner.
The paginate()
method retrieves a specified number of records (default is 15) and then displays them in a paginated view. The user can then navigate through the pages to view the remaining records.
One advantage of using the paginate()
method is that it can improve the user experience by displaying the data in a paginated view. Additionally, the paginate()
method can be faster than the chunk()
method since it requires only one database query.
However, one disadvantage of using the paginate()
method is that it can be memory-intensive when working with large datasets. Here’s an example of using the paginate
method:
$users = DB::table('users')->paginate(15);
Choosing Between Chunk and Paginate Methods
When deciding between the chunk()
and paginate()
methods, it’s important to consider the specific use case. If you need to perform an operation on each record in the result set, or if you need to process the data in batches, then the chunk()
method may be the best approach. On the other hand, if you need to display a large amount of data to the user in a user-friendly manner, then the paginate()
method may be the better option.
In addition to the use cases mentioned above, the size of the dataset also plays a role in determining which method to use. chunk()
is more suitable when dealing with large datasets since it retrieves records in smaller batches, preventing memory issues. On the other hand, paginate()
is more suitable when dealing with smaller datasets since it retrieves all records in a single query.
Another factor to consider is the performance of the application. If performance is critical, then chunk()
may be a better option since it requires fewer resources when compared to paginate()
. However, if the application needs to display data to the user, then paginate()
may be the better option.
Conclusion
In conclusion, the chunk()
and paginate()
methods in Laravel provide different approaches for retrieving and processing large amounts of data from your database. The chunk()
method is best used when you need to process the data in batches, while the paginate()
method is best used when you need to display the data in a paginated view. By understanding the differences between these methods, you can choose the best approach for your specific use case and improve the performance of your Laravel application.
I hope this article has shown you the difference between the chunk and paginate methods in Laravel. If you enjoyed reading this post and would like me to write an article on a specific topic, please send an email to info@codewithdary.com.