• 5 min read
Difference between insert() and create() in Laravel
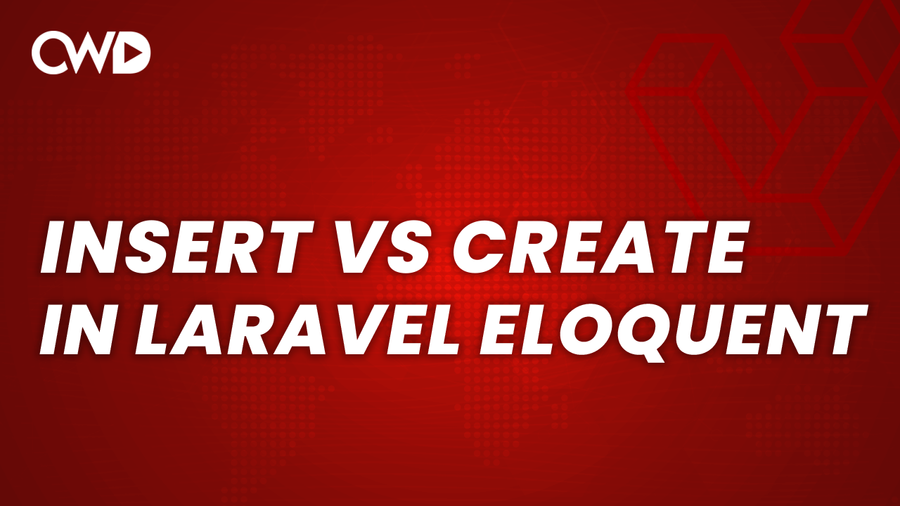
Laravel is a popular PHP web application framework that comes with a powerful ORM called Eloquent. Eloquent provides an easy and elegant way to interact with your database using object-oriented syntax. Eloquent ORM provides two methods to add new records to the database: insert()
and create()
. Although they may seem similar, they have some key differences in terms of usage and functionality.
The Basics
Both insert() and create() are methods used to insert data into your database. However, they differ in terms of how they handle the data being inserted.
Insert Method
The insert()
method is a static method that is used to insert a new record into a database table. It is useful when you need to insert multiple rows at once. This method will execute a single insert statement, which is often faster than inserting each row individually. Also, you can specify additional SQL clauses, like ON DUPLICATE KEY UPDATE, to handle errors and conflicts.
It is used to insert one or more records into the database in a single query. It takes an array of key-value pairs where the keys represent the columns of the table and the values represent the values to be inserted into those columns.
For example, let’s say we have a users
table with name
, email
, and password
columns. We can use the insert()
method to add a new user to the table as follows:
User::insert([
['name' => 'John Doe', 'email' => 'johndoe@example.com'],
['name' => 'Jane Doe', 'email' => 'janedoe@example.com']
]);
One thing to note about the insert()
method is that it does not return the ID of the inserted record. This means that if you need to retrieve the ID of the newly inserted record, you will need to make a separate call to the database.
Insert does not add timestamps
The insert()
method does not add the created_at
and updated_at
columns because it is a static method that is used to insert one or more records into the database in a single query.
Since the created_at
and updated_at
columns are automatically managed by Laravel, they do not need to be included in the array of values passed to the insert()
method. If you want to set these columns manually, you can do so using the update()
method after the record has been inserted. Alternatively, you can use the create()
method, which automatically sets the created_at
and updated_at
columns for you.
Create Method
The create()
method, on the other hand, allows you to create a new record and persist it to the database in a single call. It takes an array of key-value pairs just like the insert()
method, but it returns the newly created model instance.
For example, let’s say we have a User
model with name
, email
, and password
attributes. We can use the create()
method to add a new user to the database as follows:
$user = User::create([
'name' => 'John Doe',
'email' => 'johndoe@example.com',
'password' => bcrypt('password'),
]);
The create()
method also automatically sets the created_at
and updated_at
timestamps for the newly created record.
Key Differences
The main difference between the insert()
and create()
methods can be summarized as follows:
- The
insert()
method is used to insert a new record into a database table, while thecreate()
method creates a new record and persists it to the database in a single call. - The
insert()
method does not return the ID of the inserted record, while thecreate()
method returns the newly created model instance. - The
create()
method automatically sets thecreated_at
andupdated_at
timestamps for the newly created record, while theinsert()
method does not.
Use Cases
Both insert()
and create()
methods have their own use cases in Laravel Eloquent ORM.
The insert()
method is useful when you need to insert multiple records at once. For instance, if you need to import a large dataset into your application, you can use the insert()
method to add all the records at once, instead of making multiple calls to the database.
On the other hand, the create()
method is useful when you need to create a single record and persist it to the database in a single call. This is often the case when you are creating a new user account or adding a new item to a shopping cart.
Conclusion
In conclusion, both insert()
and create()
methods have their own use cases in Laravel Eloquent ORM. If you need to insert multiple records at once, insert()
is the better option. However, if you need to create a single record and persist it to the database in a single call, create()
is the way to go.
By understanding the differences between these two methods, you can choose the one that best suits your needs and avoid any potential issues that may arise from using the wrong method.