• 4 min read
Dynamic Where Clauses in Laravel: Advantages, Disadvantages, and Examples
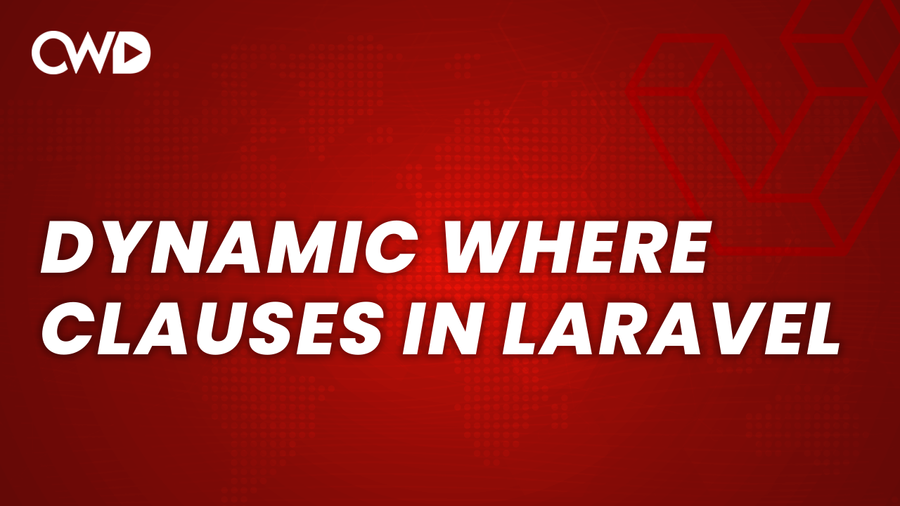
When working with Laravel, you may have come across the concept of a dynamic where clause. This feature is often overlooked and not talked about in Laravel discussions, but it can be a powerful tool in your development arsenal. In this post, we will explore the advantages and disadvantages of using the dynamic where clause in Laravel, and we will use the examples of whereEmail and whereName to illustrate its functionality.
Advantages of the Dynamic Where Clause
One of the primary advantages of using the dynamic where clause in Laravel is its flexibility. With this feature, you can dynamically construct your SQL queries based on the conditions you specify. This can be especially useful when working with complex queries that require a lot of conditional statements.
Another advantage of the dynamic where clause is that it allows you to easily build queries that are customizable by the end user. For example, if you are building a search feature for your application, you can use the dynamic where clause to allow users to search for specific attributes like name or email address.
The dynamic where clause can also help you write concise code. Instead of writing multiple if statements to check for different conditions, you can use the dynamic where clause to construct your query in a single line of code.
Disadvantages of the Dynamic Where Clause
One potential disadvantage of using the dynamic where clause is that it can lead to code that is difficult to maintain. When you are constructing complex SQL queries on the fly, it can be easy to create code that becomes hard to read and understand over time. Additionally, if you are not careful, you may end up with queries that are vulnerable to SQL injection attacks.
Another disadvantage of the dynamic where clause is that it can be slower than constructing queries manually. This is because the dynamic where clause requires Laravel to parse the conditions you specify and generate the appropriate SQL on the fly. In some cases, this can result in slower query execution times.
However, there are ways to mitigate these disadvantages. For example, you can use Laravel’s query builder to construct your queries in a more structured manner, which can make them easier to read and maintain. Additionally, you can use Laravel’s query logging feature to identify slow queries and optimize them for better performance.
Examples
To illustrate how the dynamic where clause works, let’s take a look at two examples: whereEmail
and whereName
. These methods allow you to search your database for records based on a user’s email address or name, respectively.
Here is an example of how you might use the whereEmail method to search for users with a specific email address:
$user = User::whereEmail('info@codewithdary.com')->first();
In this example, we are using the dynamic where clause to construct a query that searches for a user with the email address “info@codewithdary.com”. The ‘first()’ method returns the first user that matches this criteria.
Similarly, you can use the whereName method to search for users by name:
$users = User::whereName('Dary')->get();
In this example, we are using the dynamic where clause to construct a query that searches for all users with the name “John”. The ‘get()’ method returns all users that match this criteria.
Conclusion
While the dynamic where clause in Laravel may not be as well-known or widely-discussed as some other features, it can be a powerful tool in your development arsenal. By using this feature, you can build flexible, customizable queries that allow your users to search for specific attributes in your database.
However, it is important to be aware of the potential disadvantages of using the dynamic where clause, such as code that is difficult to maintain and slower query execution times. As with any feature in Laravel, it is important to weigh the pros and cons carefully and use the dynamic where clause judiciously. By doing so, you can take advantage of its benefits and avoid its pitfalls to build better, more efficient applications.
I hope this article has shown you the power of dynamic where clauses in Laravel. If you enjoyed reading this post and would like me to write an article on a specific topic, please send an email to info@codewithdary.com.