• 3 min read
How does the insertGetId() method in Laravel works?
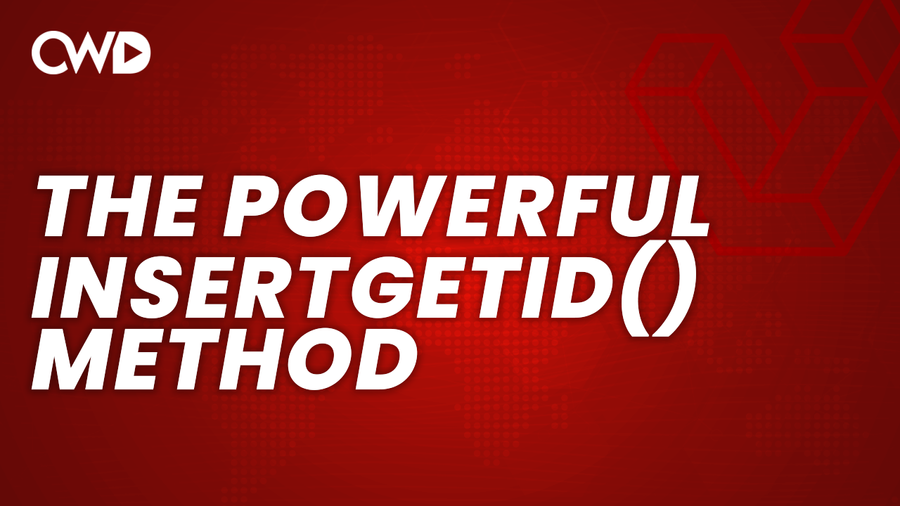
So you’re using the Laravel PHP framework and you’ve stumbled upon the insertGetId()
method. This sweet little method lets you insert a record into a database table and get back the ID of the record you’ve just inserted. In this blog post, we’re gonna go behind the scenes and find out how this method works in both the query builder and Eloquent ORM.
Using insertGetId() with the Query Builder
So, when you’re building a query, you can use the insertGetId()
method to add a new record to your database table and get the ID of the new record in one fell swoop. Here’s how it works: First, the insert()
method is used to add the new record to your table.
Then, the insertGetId()
method is used to get the ID of the new record. This method grabs the ID of the last record that was added to your database connection. That way, you can get all the info you need in just one step, without having to run a bunch of queries or write any crazy SQL statements. It’s a real time-saver and makes your code way more efficient.
$query = DB::table('posts')->insertGetId([
'user_id' => auth()->id(),
'title' => 'Insert through the insertGetId',
'slug' => 'insert-through-the-insertgetid',
'description' => 'description',
'status' => true,
]);
In the example above, the insertGetId()
method inserts a new record into the posts
table with the name and email fields set. The ID of the inserted record is then stored in the $query
variable.
Using insertGetId() with Eloquent ORM
Eloquent is a powerful tool that simplifies the process of inserting rows and retrieving their IDs. One key feature is the insertGetId()
method, which creates a new instance of the model and sets its attributes using the provided data array.
Here’s an example of using insertGetId()
with Eloquent ORM:
$query = Post::insertGetId([
'user_id' => auth()->id(),
'title' => 'Insert through the insertGetId',
'slug' => 'insert-through-the-insertgetid',
'description' => 'description',
'status' => true,
]);
In the example above, insertGetId()
creates a new Post
instance and sets its attributes using the data array. The ID of the inserted record is then stored in the $query
variable.
Conclusion
The insertGetId()
method in Laravel makes it easy to insert a record into a database table and retrieve the inserted record’s ID in a single step. Behind the scenes, the method uses different approaches in the query builder and Eloquent ORM to achieve the same result. Understanding how this method works can help you write more efficient and effective code in your Laravel applications.
I hope this article has shown you how to use the insertGetId()
method in Laravel. If you enjoyed reading this post and would like me to write an article on a specific topic, please send an email to info@codewithdary.com.