• 4 min read
How to Effortlessly Seed Your Database Seeders Using a JSON File
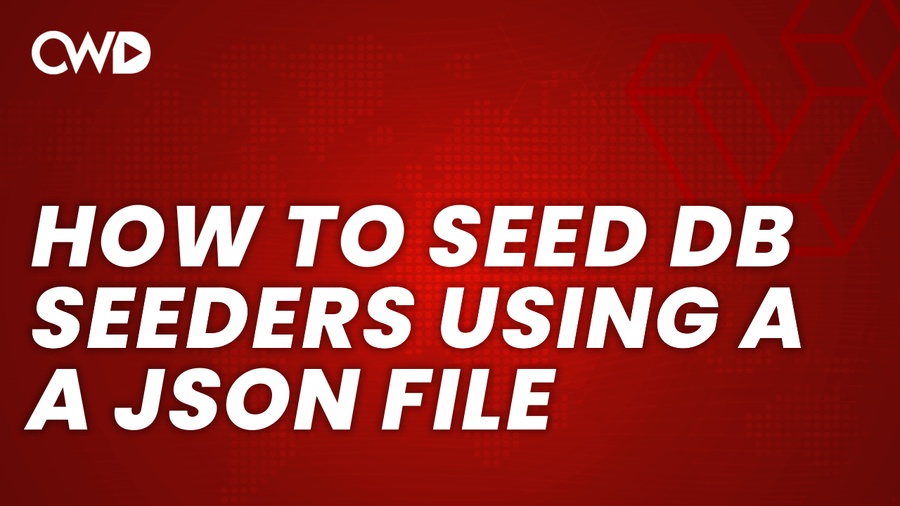
In my new course, Mastering Laravel 10 Query Builder, Eloquent & Relationships, I’ve added an episode where I recommend using a JSON file in your database seeders instead of adding an array. When building a project, you sometimes need to add data to the database to test things out. However, doing this manually can be a huge pain. Fortunately, Laravel provides seeders to help. In this blog post, we’ll show you how to use a JSON file to seed your database using seeders.
What are Seeders?
Seeders are used to populate the database with data. Laravel seeders are PHP classes that contain a run()
method that inserts data into the database. Seeders are particularly useful for testing the functionality of the application, as they allow developers to populate the database with dummy data quickly and easily.
If you have used a Seeder before, you most likely have used an array inside of your seeder. Using a JSON file to seed database seeders provides several benefits over using an array:
- Easy to maintain: JSON files are easy to read and write, making it simple to update the data being seeded.
- Standardized format: JSON is a standardized format that can be used across different programming languages and technologies.
- Improved performance: Using a JSON file to seed a database seeder can improve performance by reducing the number of queries required to insert data into the database.
Using a JSON File to Seed Database Seeders
Before we can begin seeding our database with data, we must first generate our database seeder. This step is essential to ensure that the data we insert into the database is done so correctly and efficiently.
php artisan make:seeder PostSeeder
This will generate a new PostSeeder class inside the database/seeds
directory. First, create a new directory named JSON inside the database
directory. Then, create a .json file with a name that matches the model you will use to persist the data. An example of a JSON file is shown below:
[
{
"title": "Post One through Seeder",
"slug": "post-one-through-seeder",
"excerpt": "Just a Excerpt..",
"description": "Just a Description..",
"is_published": true,
"min_to_read": 4
},
{
"title": "Post Two through Seeder",
"slug": "post-two-through-seeder",
"excerpt": "Just a Excerpt..",
"description": "Just a Description..",
"is_published": true,
"min_to_read": 4
}
]
Inside the run()
method of the PostSeeder
class, we should read the contents of the JSON file using either the file_get_contents()
function or the File()
facade:
use Illuminate\Support\Facades\File;
$json = File::get('database/json/posts.json');
Then, we need to convert the JSON data to an array using the json_decode()
function:
$posts = json_decode($data, true);
When working with Laravel, I prefer to use collections because they provide access to helpful methods. To wrap a value inside a collection, use the collect()
method.
$posts = collect(json_decode($json));
In this scenario, we have a collection of records that we want to insert into a database. While a basic foreach
loop could accomplish this task, we can leverage the power of Laravel’s collection methods to streamline the process.
Instead of relying on a traditional foreach
loop, we can chain the each
method onto our collection. This allows us to iterate through each record in the collection and insert them into the database one by one.
By using this method, we can reduce the amount of boilerplate code needed to complete the task, making our code more concise and easier to read. Not only that, but the each
method also provides additional functionality that we can take advantage of, such as filtering and sorting.
So in summary, while a basic foreach
loop can get the job done, using Laravel’s collection methods can make the process more efficient and easier to manage in the long run.
$posts->each(function ($post) {
Post::insert([
"title" => $post->title,
"slug" => $post->slug,
"excerpt" => $post->excerpt,
"description" => $post->description,
"is_published" => $post->is_published,
"min_to_read" => $post->min_to_read
]);
});
Conclusion
Seeding a database with data is an essential part of building an application. Laravel seeders provide a convenient way to automate this process. Using a JSON file to seed a seeder can make the process even easier and more efficient. By following the steps outlined in this blog post, you can quickly and easily seed your database with data from a JSON file.
I hope this article showed you how to easily use a JSON file to seed your database seeders. If you enjoyed reading this post and would like me to write an article on a specific topic, please send an email to info@codewithdary.com.