• 4 min read
Can You Make Hidden Attributes Visible in Laravel?
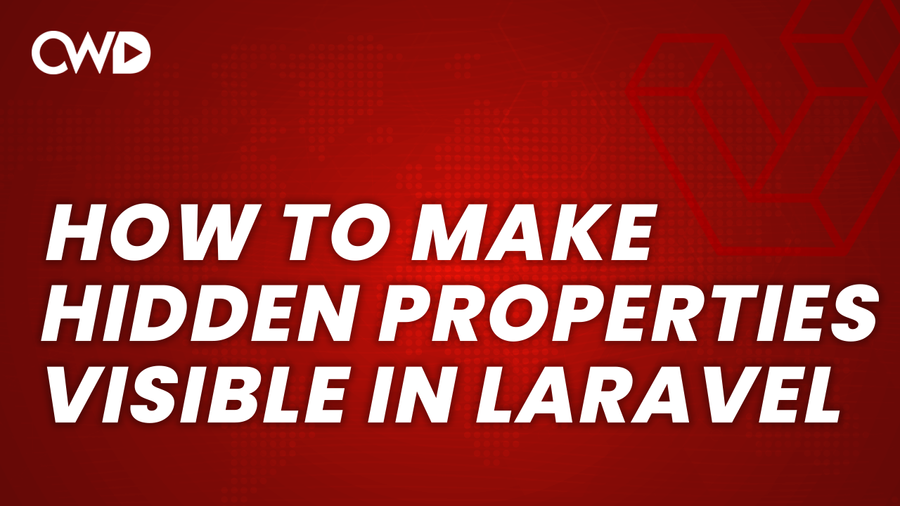
Laravel is a powerful and popular PHP framework that provides many features to make web development easier and more efficient. One of these features is the ability to hide certain attributes in a model, which can be useful for security or privacy reasons. However, there may be situations where you need to access these hidden attributes. In this blog post, we will discuss the makeVisible()
method in Laravel, which allows you to make hidden attributes visible.
The User Model and Hidden Properties
To demonstrate the makeVisible method, let’s consider the User model in Laravel. By default, the User model comes with several hidden properties, such as the users password
, and remember_token
.
These properties are hidden to prevent sensitive information from being exposed to unauthorized users. This is a good practice to ensure data security. You are completely free to add more hidden properties. Let’s also include the user’s nickname here.
/**
* The attributes that should be hidden for serialization.
* @var array<int, string>
*/
protected $hidden = [
'nickname',
'password',
'remember_token',
];
However, there may be times when you need to access these hidden properties. For example, you may want to display the user’s password hash for debugging purposes. In this case, you can use the makeVisible()
method to make the hidden properties visible.
The makeVisible()
method is a method on the Eloquent Model class in Laravel. It is used to make specified attributes visible for the model instance. In other words, it allows you to temporarily override the hidden attribute setting for a specific instance of a model.
Creating a Controller with the show Method
To use the makeVisible method, we need to create a controller with a show method that retrieves a user and makes the hidden properties visible. Here is an example of how to do this:
<?php
namespace App\Http\Controllers;
use App\Models\User;
class UserController extends Controller
{
public function show(User $user)
{
$user->makeVisible(['nickname', 'password']);
// Continue
}
}
In this example, we first retrieve the user with the specified ID
. We then call the makeVisible()
method on the user object, passing in an array of the hidden properties we want to make visible. In this case, we want to make the nickname
and password
properties visible.
Outputting a User with Hidden Properties Visible
Now that we have our controller and show method set up, we can output a user with hidden properties visible. Let’s see an example down below:
App\Models\User {
id: 1,
name: "Libbie Stiedemann",
nickname: "Lib",
email: "dimitri35@example.org",
email_verified_at: "2023-03-03 13:14:50",
date_of_birth: "1995-06-03 10:04:50",
created_at: "2023-03-03 13:14:50",
updated_at: "2023-03-03 13:14:50",
}
The response from the request will be the user object, with the nickname
and password
properties now visible. We can use this information for debugging or any other necessary purposes.
Conclusion
The makeVisible()
method in Laravel provides a simple and powerful way to make hidden attributes visible in a model. By using the makeVisible()
method in a controller’s show method, we can retrieve a user with hidden properties visible and output the information we need. This can be extremely useful for debugging or any other situations where we need access to hidden attributes.
In conclusion, the makeVisible()
method is a great feature that can help developers to debug and solve problems easily. It is important to use this method only when it is necessary and in a secure environment, since exposing sensitive data can cause security issues. Laravel is a great framework that provides many useful features, and the makeVisible method is an example of how Laravel can make web development easier and more efficient.
I hope this article has shown you how you could easily make hidden properties visible in Laravel. If you enjoyed reading this post and would like me to write an article on a specific topic, please send an email to info@codewithdary.com.