• 4 min read
Mastering Controllers and Routes in Symfony: A Comprehensive Guide
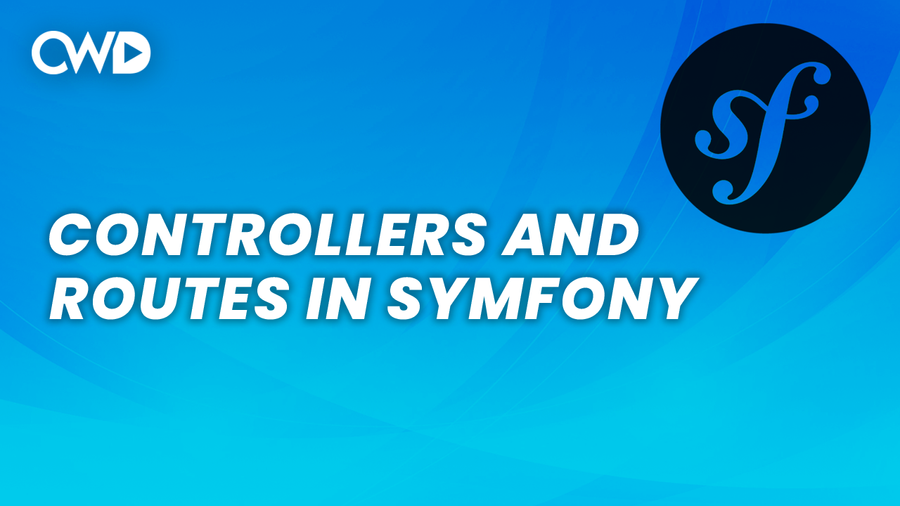
Controllers are essential in the Model-View-Controller (MVC) design pattern used in Symfony. They are classes that organize the logic of one or more routes in one place, making it easier for developers to manage and maintain their code. Controllers act as traffic cops that route HTTP requests around your application, ensuring that each request reaches the appropriate location in your codebase.
Before creating a controller, it is important to understand the configuration format
in Symfony. You have three options to choose from: YAML, XML, and PHP. YAML is simple, clean, and readable, but not all IDEs support autocompletion and validation for it. XML is autocompleted/validated by most IDEs and is parsed natively by PHP, but sometimes generates configuration considered too verbose. PHP is very powerful and allows you to create dynamic configuration with arrays or a ConfigBuilder.
It can be challenging to decide which configuration format to choose, but the good news is that each one of them has its advantages. I recommend you use the one you’re most comfortable with. In this course, we will focus on using annotations
. Annotations allow you to add metadata to your code, making it easier to read and understand.
Symfony also has a cool feature called the console command, which is similar to Laravel’s Artisan command. It enables you to create certain files through the CLI, saving you time and effort. With Symfony’s console command, you don’t need to right-click on a directory, create a new file, name it, add PHP tags, or give it a namespace and a class name. The Symfony console does all of this for you.
Before you can use the make
command, you need to pull in the Doctrine maker through Composer. Once you have pulled in the Doctrine maker, you can use the make
command to create various files such as controllers, entities, and forms. This feature is very useful, particularly for developers who want to focus on writing code and not waste time on repetitive tasks.
composer require doctrine maker
With Doctrine Maker, you can create a controller (and more) with the following command:
symfony console make:controller MoviesController
The last parameter will be the name of your class and file. In our case, it will be MoviesController
. The output will be a success
message with the path of our Controller. Controllers will be stored in the src/Controller/
directory, where a class with the name MoviesController.php
has been added.
A quick note: Keep in mind that the file name needs to be the same as the class name, otherwise you will run into errors.
The real magic of our application will happen inside the MoviesController
class, where you define routes and methods.
Right above our index()
method, you’ll see an attribute that looks like a comment. It’s actually a route attribute that defines the route for the method mentioned below it. The first parameter is the endpoint that you need to add to the URL. In our case, it will be /movies
. The name is an optional parameter that defines the name of our endpoint.
#[Route('/movies', name: 'movies')]
If you navigate to the browser and change the endpoint to 127.0.0.1:8000/movies
, you will see the default JSON response that was added inside the index()
method.
What happens if the endpoint does not exist?
This is an interesting question since it’s highly likely that you’ll try to access the wrong endpoint. If you change the endpoint inside the annotations from /movies
to /movie
, Symfony will respond with the following message:
No route found for “GET <http://127.0.0>.:8000/movies”
This error message appears because the endpoint does not exist.
What is the difference from previous Symfony versions?
If you’re using an older PHP version or possibly a newer version in the future, your annotations or attributes might look different than mine, even if you search for solutions to your routing issues online.
In previous Symfony versions, annotation methods used to look like this:
/**
* @Route("/movies", name="movies")
*/
public function index(): Response
{
//
}
controllers:
resource: ../../src/Controller/
type: annotation
The code above creates a route from the controller action methods and their route annotations.
Conclusion 🚀
We hope this article has provided you with a clear understanding of how to set up controllers and routes in Symfony.
If you enjoyed reading this post and would like us to cover a specific topic, please send an email to info@darynazar.com.