• 4 min read
Mastering the updateOrCreate() & upsert() Method in Laravel's Eloquent ORM
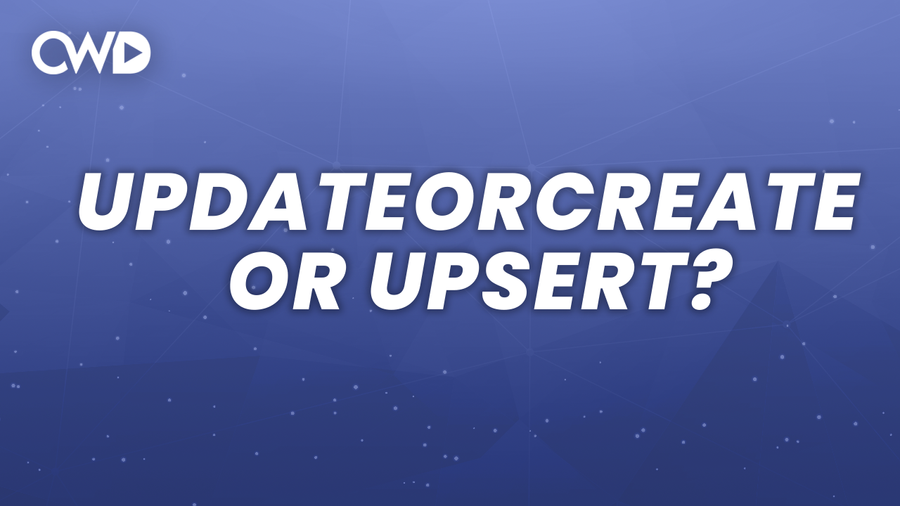
One of the key features of Laravel is its powerful object-relational mapping (ORM) system, which allows developers to interact with databases using object-oriented programming techniques. Laravel’s ORM, called Eloquent, provides a wide range of features for working with databases, including the upsert method. In this blog post, we will discuss the advantages and disadvantages of using the upsert method in Laravel Eloquent ORM and when to use it. We will also provide multiple examples to demonstrate its usage.
Advantages of Upsert Method in Laravel Eloquent ORM
The upsert method in Laravel Eloquent ORM provides many advantages to developers. It allows developers to insert or update records in a single query, which saves time and reduces the number of database transactions. This method also simplifies the code and reduces the number of lines developers need to write, making the code more readable and maintainable.
Another advantage of using the upsert method is that it enables developers to handle concurrency issues. When multiple users try to update the same record simultaneously, the upsert method solves the problem by either inserting a new record or updating an existing one, depending on the conditions specified.
Finally, he upsert method provides a more elegant solution to the problem of updating or inserting records in a database. Traditionally, developers would need to write separate queries to update existing records and insert new records. With the upsert method, developers can accomplish both tasks with a single query, making their code much more efficient.
Disadvantages of Upsert Method in Laravel Eloquent ORM
Although the upsert method provides many advantages, it also has some disadvantages. One of the main disadvantages is that it can be slower than traditional insert or update methods, especially when dealing with large datasets. The upsert method requires additional checks to determine if a record already exists, which can be time-consuming.
Another disadvantage is that the upsert method can be more complex and difficult to debug than traditional insert or update methods. Developers need to be careful when specifying conditions, as incorrect conditions can result in unexpected behavior.
When to Use Upsert Method in Laravel Eloquent ORM
Developers should consider using the upsert method in Laravel Eloquent ORM when they need to insert or update records in a database table. This method is particularly useful when dealing with large datasets or when handling concurrency issues.
Here are a few examples of when to use the upsert method:
Example 1: Updating User Information
Suppose you have a table called “users” that contains information about your website’s users. You want to update a user’s information if the user already exists in the table, or insert a new record if the user does not exist. The upsert method can be used to accomplish this task in a single query:
User::updateOrCreate(
['email' => 'john@example.com'],
['name' => 'John Doe', 'phone' => '555-5555']
);
Example 2: Inserting or Updating Product Inventory
Suppose you have a table called “products” that contains information about your product inventory. You want to insert a new record if a product does not exist, or update the existing record if it does. The upsert method can be used to accomplish this task in a single query:
Product::updateOrCreate(
['sku' => 'ABC123'],
['name' => 'Product Name', 'price' => 19.99, 'quantity' => 100]
);
Example 3: Upsert with Query Builder
In addition to using the upsert method with Eloquent models, developers can also use it with the Query Builder. This won’t be done through the updateOrCreate
method, but through the upsert
method. Here’s an example of how to use the upsert method with the Query Builder:
DB::table('users')
->upsert(
['email' => 'john@example.com'],
['name' => 'John Doe', 'phone' => '555-5555']
);
Conclusion
The upsert method in Laravel Eloquent ORM provides many advantages, including simplifying database operations, reducing the number of lines of code, and handling concurrency issues. It also has some disadvantages, including potential performance issues and increased complexity. Developers should consider using the upsert method when dealing with large datasets or handling concurrency issues. By understanding the advantages and disadvantages of the upsert method and when to use it, developers can make informed decisions about how to handle database operations in their Laravel applications. Overall, the upsert method is a powerful tool for developers, and it is worth exploring in more depth for those who are interested in building efficient and scalable web applications.
I hope this article has shown you upserting data works in Laravel. If you enjoyed reading this post and would like me to write an article on a specific topic, please email info@codewithdary.com.