• 5 min read
What is inside the tests subdirectory in Laravel?
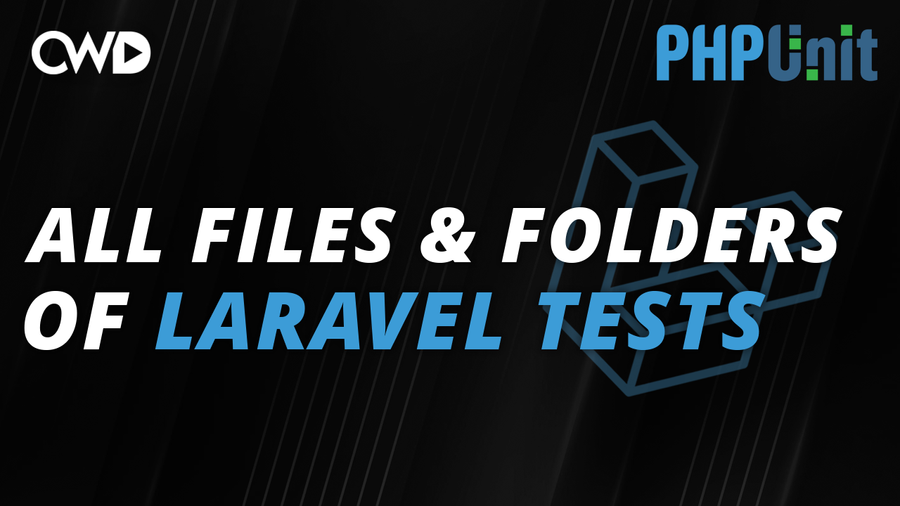
Laravel makes it very easy for developers to test their applications. But testing can be very overwhelming once you are new to it. In this article, we are going to cover the basics of testing, the files that have been added, the subdirectories that have been added, and how tests can be run inside through the CLI.
Why do developers test?
We can all agree that testing is a good thing, whether you are a developer, doctor, or fireman. In coding, there are lots of different tools that you can use for testing. The following four tools are the most popular ones right now.
Instead of setting your test environment manually, Laravel has PHPUnit integrated by default. PHPUnit
is the most popular PHP framework independent library that can be used for unit testing.
What is unit testing?
I am going a bit too fast, I’ve already mentioned unit testing
without covering unit testing
. Unit testing is a method that you can use where small pieces of code will be tested against expected results. If you are new to testing and it is completely new for you, you might wonder when you all be using it. Think about the following three situations:
- Crawling your site’s URI
- Tests on form submissions
- Tests on status codes
Testing basics
During this tutorial, you will be hearing quite some new terms that you might not have heard before. I would like to talk about the most used terms before we move into the code.
Unit tests
Unit tests verify if individual units of code work as expected.
Feature tests
Feature tests will test the way individual units work together and pass messages.
Application tests
Application tests will test the entire behavior of your application, which eventually leads into releasing a bug-free and robust software application.
Regression tests
With regression tests, you’re going to describe exactly what a user should be able to do and make sure that the application will not stop.
We will not be covering all these tests in this tutorial, but it is good to keep in mind that there are more tests that unit tests.
Directory/file structure
Laravel adds some directories and files by default in the root of your project directory. Which is awesome, because you don’t need to configure anything for your testing environment. Inside the root of your directory, you will find a directory called /tests
.
-tests
- Feature [subdirectory]
- Unit [subdirectory]
+ CreateApplication.php [file]
+ TestCase.php [file]
Subdirectories
The feature
subdirectory consists of tests that will cover the interaction between multiple units, while the unit
subdirectory consists of tests for one unit of your code.
Both subdirectories come with an ExampleTest.php
file inside of it which consists of a sample test.
Inside the ExampleTest.php
file, you will find your first basic unit test. Keep in mind that unit tests should be simple. You will see that the test_example()
test simply checks if the assert is true. The method accepts one parameter, which is true. In our case, the test will always return true
.
public function test_example()
{
$this->assertTrue(true);
}
Files
The CreateApplication.php
file isn’t a class but it is a Trait. The same Trait will be used inside the TestCase.php
file which will be the base root test that all our other tests will extend.
use CreatesApplication;
How to run tests
One of the most important things about testing is running them. You can create as many tests as you want, but you cannot test anything if it doesn’t work. Does that make sense? If you have an error inside on the test, you will be prompted with an error message and it will stop running any other test.
Laravel makes it very easy for us to run our tests through the terminal. Keep in mind that you can only perform tests from the root of your project directory. You can run your tests by performing one of the following commands:
./vendor/bin/phpunit // First method
php artisan test // Second method
// OUTPUT EXAMPLE (./vendor/bin/phpunit)
PHPUnit 9.5.8 by Sebastian Bergmann and contributors.
2/2 (100%)
Time: 00:00.200, Memory: 18.00 MB
Tests output
The output of a test might seem weird if you’re seeing it for the first time. The PHPUnit version is 9.5.8. It has been created by Sebastian Bergmann and contributors. The two dots indicate that we performed two tests, which is true because we performed the ExampleTest.php
from the Feature
and Unit
subdirectory.
PHPUnit file
Whenever you run your tests, you will be running the phpunit.xml
file from the root of your directory.
The most important piece of code is the testsuites
section, which makes sure that PHPUnit can run the ./tests/Unit
and ./tests/Feature
subdirectories.
<testsuites>
<testsuite name="Unit">
<directory suffix="Test.php">./tests/Unit</directory>
</testsuite>
<testsuite name="Feature">
<directory suffix="Test.php">./tests/Feature</directory>
</testsuite>
</testsuites>
This is the bare minimum you need to know before you start writing tests. In the next article, we will be covering how we could write tests.