• 6 min read
Understanding the Differences Between Laravels each() and foreach() Methods
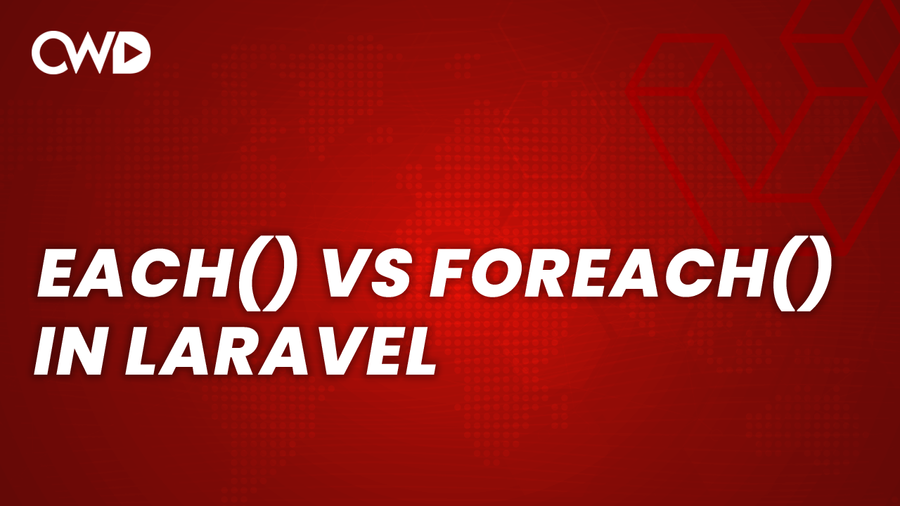
Laravel is a popular PHP framework that provides a lot of useful features and functions to help developers write efficient and clean code. Two of these functions are each()
and foreach()
. Both methods are used for looping through arrays, but there are some differences between them that we will explore in this blog post.
When to Use Each
The each()
method is used to iterate through an array and execute a callback function for each element. The main advantage of using each()
over foreach()
is that it stops iterating once the callback function returns false
. This can be useful in situations where you want to break out of a loop early, such as when you find the item you are looking for.
Here is an example of how to use each()
:
$items = [
'apple',
'banana',
'orange',
'pear',
];
collect($items)->each(function ($item, $key) {
echo '$key: $item';
if ($item === 'orange') {
return false;
}
});
In this example, we have an array of fruits, and we use collect()
to convert it to a Collection. We then call each()
on the Collection and pass in a callback function that echoes out the key and value of each element. If the element is ‘orange’, we return false
, which stops the loop from continuing.
One of the main advantages of using each()
is that it can help improve performance by breaking out of a loop early. This is because each()
only continues iterating until the callback function returns false
. If you have a large array and are searching for a specific item, using each()
can help you find the item faster by stopping the loop as soon as it is found.
However, it’s important to note that each()
can also make your code harder to read and understand, especially if you are not familiar with the each()
method. This is because each()
is not as commonly used as foreach()
, which means that other developers may have a harder time understanding your code if you use each()
.
When to Use foreach
The foreach()
method is used to iterate through an array and execute a block of code for each element. Unlike each()
, foreach()
will iterate through the entire array, regardless of whether the block of code returns true
or false
.
Here is an example of how to use foreach()
:
$items = [
'apple',
'banana',
'orange',
'pear',
];
foreach ($items as $key => $item) {
echo '$key $item';
}
In this example, we use a traditional foreach()
loop to iterate through the array and echo out the key and value of each element.
One advantage of using foreach()
over each()
is that it is a more common and widely used method for iterating through arrays. This means that other developers are more likely to be familiar with foreach()
and will have an easier time understanding your code if you use it.
However, foreach()
does not offer the same level of flexibility as each()
. This means that if you need to break out of a loop early, foreach()
may not be the best choice. In situations where you need more flexibility, each()
may be a better option.
Advantages and Disadvantages
As we’ve already mentioned, one advantage of using each()
is that it can help improve performance by breaking out of a loop early. It’s important to note, however, that the performance benefits of using each()
may not always be significant. If you are working with a small array, the difference in performance between each()
and foreach()
may be negligible.
On the other hand, one of the main advantages of using foreach()
is that it is a more common and widely used method for iterating through arrays. This means that other developers are more likely to be familiar with foreach()
and will have an easier time understanding your code if you use it.
One disadvantage of using each()
is that it can make your code harder to read and understand, especially if you are not familiar with the each()
method. This is because each()
is not as commonly used as foreach()
, which means that other developers may have a harder time understanding your code if you use each()
.
Another disadvantage of using foreach()
is that it does not offer the same level of flexibility as each()
. This means that if you need to break out of a loop early, foreach()
may not be the best choice. In situations where you need more flexibility, each()
may be a better option.
Example Advantage
Another advantage of using foreach()
over each()
is that it can be used to iterate through multiple arrays simultaneously. This can be useful in situations where you have two arrays that are related to each other, such as a list of names and a list of ages.
Here is an example of how to use foreach()
to iterate through multiple arrays:
$names = [
'John',
'Mary',
'Bob',
];
$ages = [
25,
30,
40,
];
foreach ($names as $key => $name) {
$age = $ages[$key];
echo '$name is $age years old';
}
In this example, we have two arrays, $names
and $ages
, that are related to each other. We use a foreach()
loop to iterate through the $names
array and get the corresponding age from the $ages
array using the $key
variable. We then echo out the name and age of each person.
Conclusion
In conclusion, both each()
and foreach()
are useful methods for looping through arrays in Laravel. each()
offers more flexibility and performance benefits, but can be harder to read and understand. foreach()
is a more common and widely used method that is easier to read and understand, but does not offer the same level of flexibility as each()
. It is important to understand the differences between these two methods and choose the one that best fits your specific use case.
I hope this article has shown you the differences between Larvel its each and foreach methods. If you enjoyed reading this post and would like me to write an article on a specific topic, please send an email to info@codewithdary.com.