• 4 min read
10 Must-Know Column Modifiers for Laravel Migrations
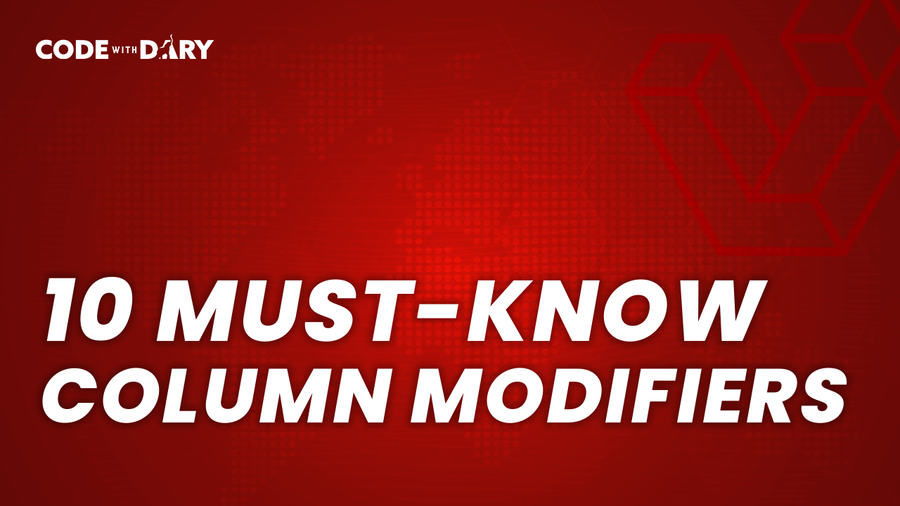
What’s up developers and welcome back to a new blog post (it’s been a while). Today, we’re going to explore 10 column modifiers that you must know when working with Laravel migrations. These modifiers will help you customize and optimize your database columns to meet your specific requirements.
It’s important to note that you won’t always need to use all of these column modifiers in every Laravel migration. However, having knowledge of these modifiers can greatly enhance your ability to customize and optimize your database structure when needed. It’s good to have these modifiers in your toolkit for future reference and use.
1. after()
The after()
column modifier is useful when you want to add an additional column to an existing table and specify its position next to another column. If you want to place the email
column after the name
column you can use the following snippet:
$table->string('email')->after('name');
2. comment()
The comment()
column modifier lets you add a descriptive comment to a column. This can be useful for documenting the purpose or additional details about a specific column. For instance, if you want to add a comment to the title
column in the posts
table, you can do it like this:
$table->string('title')->comment('The title of the post');
3. default()
Personally, one of the column modifiers I use the most is default()
for defining a default value for boolean columns. It allows me to set a default value of either true
or false
for a boolean column, ensuring that the column is always assigned a value if no explicit value is provided during insertion.
$table->integer('is_published')->default(false);
4. invisible()
The invisible()
column modifier hides the column from query results. This can be useful when you have columns that are internally used but not intended to be shown in the output.
$table->string('password')->invisible();
5. always()
The always()
column modifier ensures that a column is included in SELECT *
queries, even if you explicitly specify certain columns to select. This can be handy when you have essential columns that you always want to retrieve. For example, to make the id
column always selected in the posts
table, use this code:
$table->bigIncrements('id')->always();
6. isGeometry()
The isGeometry()
column modifier marks a column as a spatial geometry column. This is used for storing geometric data like points, lines, or polygons. To make the location
column a geometry column in the city
table, you can do the following:
$table->geometry('location')->isGeometry();
7. virtualAs()
The virtualAs()
column modifier allows you to create a virtual (computed) column based on an expression. This column won’t be physically stored in the database but can be useful for calculations or aggregations. Let’s say you want to create a virtual column named full_name
in the users
table that concatenates the first_name
and last_name
columns:
$table->string('full_name')->virtualAs('CONCAT(first_name, " ", last_name)');
8. useCurrent()
The useCurrent()
column modifier sets the column value to the current timestamp when a new row is inserted. This is commonly used for columns like created_at
or updated_at
. To set the created_at
column to the current timestamp in the posts
table, use the following code:
$table->timestamp('created_at')->useCurrent();
9. charset()
The charset()
column modifier sets the character set for a string column. This is helpful when working with multilingual applications or different character sets. For example, to set the name
column’s character set to UTF-8 in the users
table, you can use this code:
$table->string('name')->charset('utf8');
10. storedAs()
The storedAs()
column modifier allows you to store a computed (generated) column based on an expression. This column will be physically stored in the database. Let’s say you want to create a stored column named total_price
in the orders
table that calculates the product of quantity
and price
:
$table->decimal('total_price', 8, 2)->storedAs('quantity * price');
Conclusion
In conclusion, understanding and utilizing column modifiers in Laravel migrations can significantly enhance your ability to customize and optimize your database structure. We explored 10 must-know column modifiers that allow you to add columns, specify their positions, add comments, set default values, hide columns, include columns in select queries, mark columns as spatial geometry, create virtual columns, set current timestamps, define character sets, and store computed columns.
By incorporating these modifiers into your Laravel migrations, you can tailor your database according to your specific requirements and improve the overall efficiency of your application.
I hope this article has shown you 10 cool column modifiers in Laravel. If you enjoyed reading this post and would like me to write an article on a specific topic, please send an email to info@codewithdary.com.