• 2 min read
Difference Between find() vs findOrFail() in Laravel
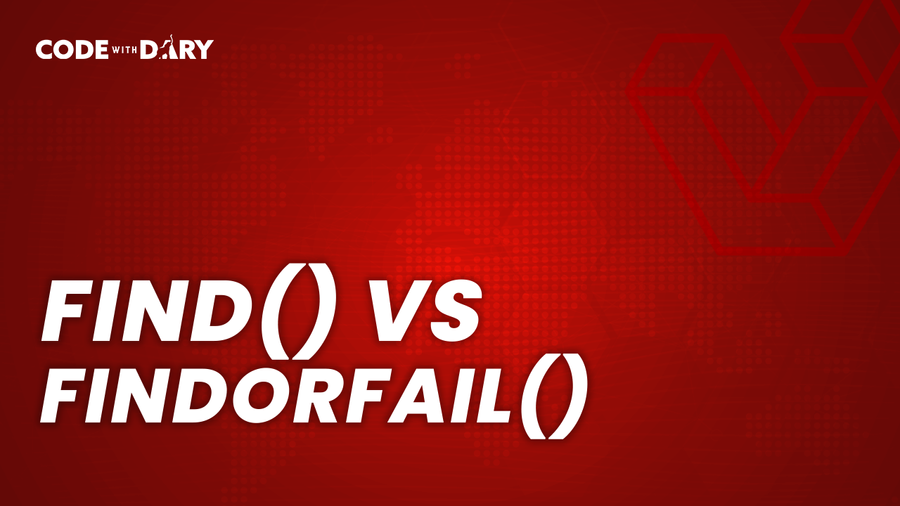
When you’re working with Laravel, you might come across the find
and findOrFail
methods when querying the database. Both of these methods are used to retrieve a record from the database, but there is an important distinction between them.
The find
Method
The find
method is really easy to use and probably one of the first methods you’ll learn when working with Eloquent. It’s used to get a record by its primary key. Just pass the primary key value as an argument, and the method will give you the matching record if it exists. If the record isn’t found, it will return null
.
Here’s an example of how you can use the find
method:
public function show($id)
{
return User::find($id);
}
The findOrFail
Method
On the other hand, the findOrFail
method also retrieves a record by its primary key. However, if the record is not found, it throws a ModelNotFoundException
exception instead of returning null
.
Here’s an example of using the findOrFail
method:
public function show($id)
{
return User::findOrFail($id);
}
By using the findOrFail
method, you can easily handle cases where finding a specific record is crucial and warrants an exception if not found.
The findOrFail
method in Laravel is useful when you want to explicitly handle the case when a record is not found. By throwing an exception, it allows you to catch and handle the error gracefully, rather than returning null
and potentially causing unexpected behavior in your application.
Conclusion
In conclusion, both the find
and findOrFail
methods handle cases when a record is not found in slightly different ways. The find
method gives you null
, while the findOrFail
method throws an exception.
By using these modifiers in your Laravel migrations, you can customize your database to fit your specific needs and make your application more efficient.
I hope this article has cleared up the distinction between the find
and findOrFail
methods in Laravel. If you liked what you read and want me to write about a particular topic, just shoot me an email at info@codewithdary.com.