• 3 min read
How to Sort Collections in Laravel
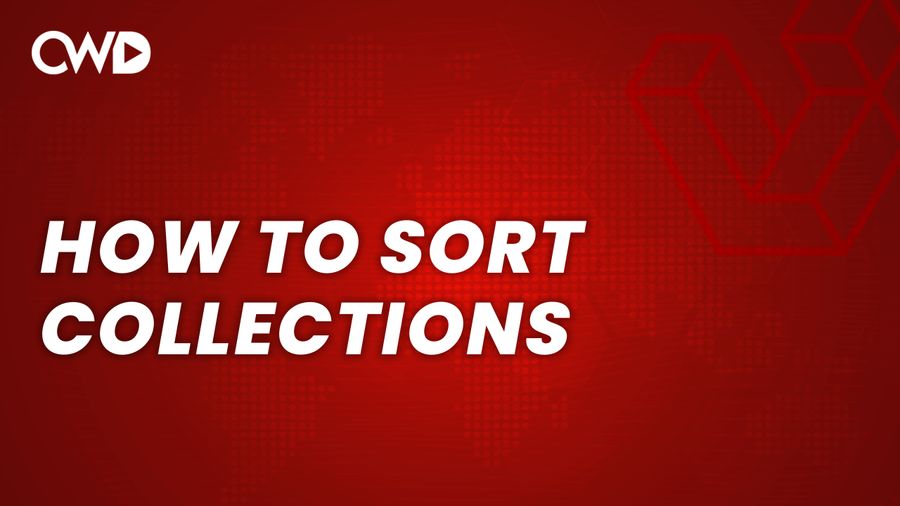
When working with collections in Laravel, it’s important to know how to sort them to better organize and display data. Laravel offers three main methods to sort collections: orderBy
, latest
, and oldest
. Each of these methods has its own strengths and use cases, and selecting the right method can make a big difference in the efficiency and readability of your code.
orderBy
The orderBy
method is used to sort a collection based on one or more attributes. It can sort the collection in ascending or descending order, and it can handle multiple sorting criteria. For example, to sort a collection of users by their name in ascending order, you can use the following code:
public function index()
{
$posts = Post::orderBy('is_published')->get();
return view('blog.index')->compact('posts');
}
You can also use the orderBy
method to sort by multiple attributes, such as sorting a list of products by their price and name. Here’s an example:
public function index()
{
$posts = Post::orderBy('is_published')->orderBy('title')->get();
return view('blog.index')->compact('posts');
}
The problem with the orderBy method is that many developers use it incorrectly. This is because it is not the most effective method when sorting based on the updated_at or created_at column. Here is an example:
public function index()
{
$posts = Post::orderBy('created_at', 'desc');
return view('blog.index')->compact('posts');
}
When you need to sort based on the updated_at
or created_at
columns, I recommend using either the latest
or oldest
method.
latest and oldest
The latest
and oldest
methods are used to sort a collection by their creation date. latest
sorts the collection by the most recently created items, while oldest
sorts the collection by the oldest created items. For example, to sort a collection of blog posts by their creation date in descending order, you can use the following code:
$posts = Post::latest()->get();
public function index()
{
// latest()
$posts = Post::latest()->get();
// oldest()
$posts = Post::oldest()->get();
return view('blog.index')->compact('posts');
}
Both latest
and oldest
can also take an optional argument to specify the column by which to sort the collection. For example, to sort a collection of users by their updated date in ascending order, you can use the following code:
$posts = Post::oldest('updated_at')->get();
Sorting Relationships
When working with relationships, you can also use these sorting methods to sort related models. For example, if you have a User
model with a hasMany
relationship to a Post
model, you can sort the related posts using the latest
and oldest
methods like this:
$user = User::find(1);
$posts = $user->posts()->latest()->get();
Conclusion
Sorting collections in Laravel is a powerful way to organize and display data. By using the orderBy
, latest
, and oldest
methods, you can easily sort collections based on different criteria. Whether you need to sort a collection by a specific attribute or by their creation date, Laravel has you covered. Additionally, sorting relationships with these methods can help you to present related data in a more meaningful way, improving the user experience of your application. So, when working with collections in Laravel, don’t forget to leverage the power of these sorting methods to make your code more efficient and readable.
I hope this article has shown you the difference between the orderBy, latest and oldest methods in Laravel. If you enjoyed reading this post and would like me to write an article on a specific topic, please send an email to info@codewithdary.com.