• 5 min read
Why is Type Hinting Extremely Important in PHP?
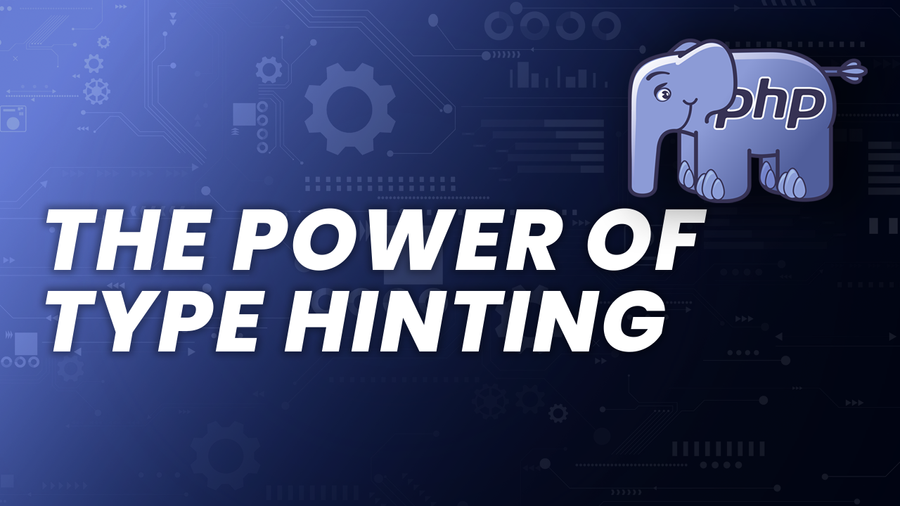
Type hinting is a programming feature that allows developers to specify the data types of variables, arguments, and return types in functions or methods. It is a feature that has been introduced in many programming languages in recent years, including PHP, Python, and Java. In this blog post, we will discuss why type hinting is important, the advantages and disadvantages of using it, how we can implement it in our code, and see some examples.
Advantages of Type Hinting
One of the main advantages of type hinting is that it helps to catch programming errors early. By specifying the data type of a variable or argument, the compiler or interpreter can detect any mismatches between the declared type and the actual type of the data. This can help prevent bugs and make the code more robust and reliable.
Another advantage of type hinting is that it can improve code readability and maintainability. By specifying the data types of variables and arguments, other developers can easily understand the purpose and usage of the code. This can make it easier to maintain the code and improve its functionality over time.
Disadvantages of Type Hinting
One of the main disadvantages of type hinting is that it can make the code more rigid and less flexible. If a data type is specified, then the code can only accept values of that type, which can limit the functionality of the code. This can be particularly problematic when working with complex data structures or when trying to integrate with other systems or libraries.
Another disadvantage of type hinting is that it can make the code more verbose and harder to read. If every variable and argument is explicitly typed, then the code can become cluttered and difficult to understand. This can be particularly problematic when working with large codebases or when collaborating with other developers.
Implementing Type Hinting in PHP
In PHP, the strict_type
declaration is used to enforce type hinting for all function arguments and return values. When strict_type
is enabled, PHP will throw an error if there is a type mismatch between the declared type and the actual type of the data.
To enable strict_type
in PHP, you can add the following line at the beginning of your script:
<?php declare(strict_types=1);
Type hinting in PHP is implemented using the type
keyword, which allows developers to specify the data type of a variable, argument, or return value. For example, the following code snippet demonstrates how to use type hinting for a function that takes a string argument:
function stringLength(string $str) : int {
return strlen($str);
}
In this example, the string
keyword is used to specify the data type of the $str
argument.
Type Hinting in PHP vs Other Programming Languages
Type hinting is a feature that is available in many programming languages, including Python, Java, and C#. Each language has its own syntax and conventions for type hinting, but the basic principles are the same. In general, strongly typed languages like Java and C# tend to have more strict type hinting requirements, while dynamically typed languages like Python and PHP tend to be more flexible.
For example, Python allows type hints to be included in the function definition or as inline comments, but it does not enforce their usage. Java, on the other hand, requires all variables and arguments to be declared with a specific type, and will throw an error if there is a type mismatch.
Example of Type Hinting in PHP
Here’s an example of how we could use type hinting in PHP to create a class that represents a car:
class Car {
private string $make;
private string $model;
private int $year;
public function __construct(string $make, string $model, int $year) {
$this->make = $make;
$this->model = $model;
$this->year = $year;
}
public function getMake() : string {
return $this->make;
}
public function getModel() : string {
return $this->model;
}
public function getYear() : int {
return $this->year;
}
}
In this example, the string
and int
keywords are used to specify the data types of the $make
, $model
, and $year
properties, as well as the arguments for the constructor and the return types for the getter methods.
Conclusion
In conclusion, type hinting is an important feature in programming that can help improve code reliability, readability, and maintainability. While there are some disadvantages to using type hinting, the benefits generally outweigh the costs, particularly in larger codebases or when working on collaborative projects. As we’ve seen, type hinting is easy to implement in PHP and other programming languages, and can be a valuable tool for any developer.
In addition, type hinting is also useful for documentation purposes, as it makes it easier for developers to understand the purpose and usage of the code. It can also help to reduce the number of bugs and errors in the code, as it makes it more difficult to accidentally misuse variables or arguments.
Overall, type hinting is a powerful tool that can help developers to write more reliable, maintainable, and efficient code. By understanding the advantages and disadvantages of type hinting, developers can make informed decisions about when and how to use it in their projects.
I hope this article has shown the advantages, disadvantages and why we type hint in PHP. If you enjoyed reading this post and would like me to write an article on a specific topic, please send an email to info@codewithdary.com.